Quants interview§
At least once in your life you have to be interviewed by a team of quants. You won’t probably love that experience but is something that everyone should face, to prove that you are now an adult.
I was interviewed by the XVA quants of Santander Bank, January of this year. I know almost nothing about how to evaluate derivatives and even about what they are so I had really low expectations, but people told me that they will make math questions more than financial ones. Knowing that, I have prepared tons of probability problems (as much as I can in 2 days), 2 of them I want to share with you.
Math problems§
These are problems that I found looking in the Internet and I think that they are beautiful.
Circle points§
If you take 3 random points into a circle, What is the probability of them staying into the same semicircle?
Let’s take 2 random points into a circle, they will always be into the same semicircle, so define the shortest distance between them as \(a\). The third point now have both, the semicircle defined from the first point to the center that contains the second point and the semicircle defined from the second point to the center that contains the first point, allowed to be inside. We can understand it better looking at the diagram:
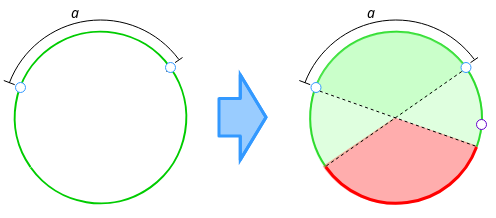
If we take a circle of length 1, \(a\) is going to be between 0 and 0.5 with 100% of probability and then we need our third point to stay outside of the region of length \(a\). So the expected value is:
So it is:
You can check this out with this chunk of Python code (which is pretty slow, but gives a fast approximation):
from random import random # Bad module - function naming
def simulate(N):
hits = 0
for i in range(N):
a = 0.5 * random()
x = random()
if x > a:
hits += 1
score = hits / (i+1)
print(f"{(i+1) / N:.2%} done. Current score is: {score}", end="\r")
print(f"\nPredicted: {3/4:.2}")
print(f"Difference: {abs((3/4) - score)}")
if __name__ == "__main__"
simulate(10**8)
Paper strip§
If you have a strip of paper, and you cut it twice, What is the probability of the resulting 3 strips to form a triangle?
ERROR
Be careful, the first solution is wrong. I just leave it here because sometimes (weird times) mistakes are beautiful. Correct answer is here.
This problem sounds pretty similar to the previous one but it is not exactly the same. The condition here is that any sum of a pair of sides must be lower than the other side, so let’s suppose that we start with a strip of 1 unit length. We cut it once, so we have 2 strips, one of length \(a\) and the other of length \(1-a\). The second cut will always be into the \(1-a\) length strip, as you can watch in this diagram:
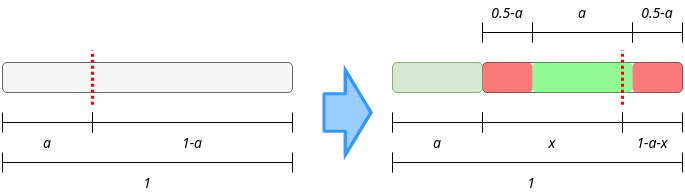
It’s easy to understand that just one half of the times our first random cut will make \(a\) lower than 0.5. Then, the second cut must be greater than \(0.5-a\) so it plus the previous are longer than 0.5 but must be lower than 0.5 itself. So we just have to calculate the expected value of this:
Solve it:
And get:
So this is the probability of the second cut to give a good piece, but to have the total probability of the problem we need to multiply by one half, which is the probability of the first cut to give a good piece. So the final solution is:
And as before, you can check it out with this code:
from random import random # Bad module - function naming
from math import log
def simulate(N):
hits = 0
for i in range(N):
a = random()
if a < 0.5:
x = (1 - a)* random():
if x > (0.5 - a) and x < 0.5:
hits += 1
score = hits / (i+1)
print(f"{(i+1) / N:.2%} done. Current score is: {score}", end="\r")
print(f"\nPredicted: {log(2) - 0.5}")
print(f"Difference: {abs(log(2) - 0.5 - score)}")
if __name__ == "__main__"
simulate(10**8)
Right way§
I have made an unnecessary assumption, and that makes me reach a wrong solution. To make this well we have to think about 2 independent cuts (let’s call them \(x\) and \(y\)), and the solution will seem pretty obvious. The conditions are:
One of the cuts must be lower than 0.5 and the other must be higher, which happens half of the times (lower - higher and higher - lower).
The absolute difference between them must be lower than 0.5.
First condition is too straightforward so let’s talk about second one. We can solve it just ignoring the \(x\)-dependence of the probability density function used in the expected value evaluated above, but to show a different way, we can define \(a\) for the highest of \(\{x,\ y\}\) and \(b\) for the lowest. Using them we can define dependent integration limits:
So this multiplied by one half, (the other condition probability) give us:
Which is the correct answer. As before, here is the code to check it:
from random import random # Bad module - function naming
from math import log
def simulate(N):
hits = 0
for i in range(N):
x, y = random(), random()
if ((x < 0.5 and y > 0.5) or (y < 0.5 and x > 0.5)) and abs(x-y) <= 0.5:
hits += 1
score = hits / (i+1)
print(f"{(i+1) / N:.2%} done. Current score is: {score}", end="\r")
print(f"\nPredicted: {0.25}")
print(f"Difference: {abs(0.25 - score)}")
if __name__ == "__main__"
simulate(10**8)
If quants ask you something like this, they will be expecting you to answer correctly in around 3-5 minutes. This problems are just for training, but let you demonstrate that you have pretty basic notions about statistics.
Financial problems§
These are the problems that make them earn money, so you can’t avoid this. Of course there’s a lot to learn about this matter but if you understand the basics, you could have a ridiculously small hope.
Black-Scholes equation§
This is the most famous equation in derivatives evaluation, and everyone must know it if he wants to be part of the business. As I said I know almost nothing about derivatives so honestly, I can’t say (barely understand) why it is that important, but I can explain how to obtain it.
Let’s suppose that you are one of the cool guys and you want to evaluate an option. If you don’t know what an option is you don’t need to, for me is just something which’s price relies on time and another thing with a random behaviour (usually a stock, I prefer to call it the underlying, feels more like a variable). So the option value can be defined like: \(V(t, S)\). Where the \(S\) stands for the underlying (the random thing). From now on you can feel that everything that we are doing is just moving the randomness deeper into equations, and that’s somehow true. But they are rich and we are not so this must be somehow true too, theoretically true.
Let’s suppose that the underlying price can be predicted using the equation of the geometric Brownian motion
Now we want to know how \(V(t, S)\) change, so we need:
Here we can say that terms on \(dt\) which’s order is higher than 1 won’t be considered. Furthermore we can replace \(dW^2\) with \(dt\) (this is also called the Itô’s lemma):
Replace \(dS\) and get the differential equation for the option value:
Now comes the financial part. We are going to build what’s called a delta-hedge portfolio. That’s what you build when you have options and you want to protect yourself from changes in their underlying price. The change in this portfolio’s value is measured like:
It’s uncertainty term (\(dW\)) has vanished, so it is a riskless portfolio. The rate of return of every riskless portfolio must be equal to the return of a zero coupon bond which is the riskless product par excellence.
And all we have to do know is equate our 2 formulas and simplify, to finally get the Black-Scholes partial differential equation:
There are many numerical methods to solve the equation and it has analytical solutions for some boundary conditions. But I won’t go further on this.
The interview§
So you have prepared this kind of problems and you feel comfortable with any medium-level math situation. You are willing to get so focused on the problems you forget you are in an interview. And you reach the quant’s space. Everyone has his lunch over the table and it seems like they spend a lot of time with their computers. No blackboards, no super-cool coffee machine, no knick-knacks to avoid your brain getting out of sugar. Not what I was expecting but let’s give it a try.
2 guys sat around me in a table into a meeting room, and asked me about my background, if I know what a CAP is (I barely knew it), and they told me that we were in a Liborg transition (we are getting into an unknown place for me) so… If Liborg’s value follows a standard normal probability distribution, how would you evaluate a CAP?.
OK. I wasn’t expecting that. I was wearing a wool sweater and I started sweating and felt my heart racing. All I had were just doubts. But what should I do.
A CAP is a product that pays the maximum of Liborg’s price minus a constant value, and zero, so:
But they had told me that the Liborg’s price follows a standard normal distribution (\(\mathcal{N}(\mu, \sigma)\)) so I just need to get the expected value:
Of course we need to keep going, even if this is currently an analytical solution. Let’s make the easiest part (this is as far as I got in the interview):
And now the hardest one:
Of course this is the same that we had before for \(K\) but for \(\mu\):
Using a new variable:
And finally putting all together:
And that’s it. I got out of the interview thinking that it was a too hard question for just a Junior interview (without any experience in derivatives evaluation) but once I got home, I thought about it more closely and didn’t find it so difficult.
I didn’t pass the interview (sad life).